Redux
Redux 初识
Redux 是状态管理工具,类似于 Vue 中的 Pinia、Vuex。
- state 对象:存放着我们管理的数据状态
- action 对象:用来描述怎么改数据
- reducer 函数:根据 action 的描述生成一个新的 state
环境搭建
Redux Toolkit(RTK):简化书写
react-redux:链接 Redux 和 React 组件的中间件
sh
npm i @reduxjs/toolkit react-redux
Redux 使用流程
store 目录结构
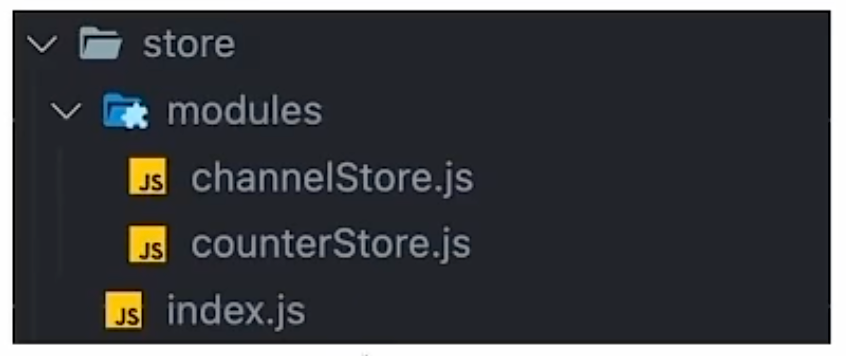
创建 Store
src/store/modules/count.js
js
import { createSlice } from '@reduxjs/toolkit';
const countStore = createSlice({
name: 'count',
// 初始值
initialState: {
count: 0
},
// 数据修改的同步方法
reducers: {
add: (state) => {
state.count += 1;
},
del: (state) => {
state.count -= 1;
},
set: (state, action) => {
state.count = action.payload;
}
}
});
const { add, del, set } = countStore.actions;
const countReducer = countStore.reducer;
export { add, del, set };
export default countReducer;
src/store/index.js
js
import { configureStore } from '@reduxjs/toolkit'
import countReducer from './modules/countStore'
const store = configureStore({
reducer: {
count: countReducer
}
})
export default store;
src/index.js
js
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
import { Provider } from 'react-redux'
import store from './store'
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<Provider store={store}>
<App/>
</Provider>
);
同步提交
src/App.js
js
import { useDispatch, useSelector } from 'react-redux'
import { add, del, set } from './store/modules/countStore'
function App () {
const { count } = useSelector((state) => state.count)
const dispatch = useDispatch()
return (
<div>
<button onClick={() => dispatch(add())}>+</button>
{count}
<button onClick={() => dispatch(del())}>-</button>
<button onClick={() => dispatch(set(0))}>-> 0</button>
</div>
);
}
export default App;
异步提交
src/store/modules/listCounter.js
js
import { createSlice } from '@reduxjs/toolkit'
import axios from 'axios'
const listStore = createSlice({
name: 'list',
initialState: {
list: []
},
reducers: {
setList: (state, action) => {
state.list = action.payload
}
}
})
const { setList } = listStore.actions
const listReducer = listStore.reducer
export default listReducer;
export { setList }
const url = 'https://geek.itheima.net/v1_0/channels'
// 异步获取方法
const fetchList = () => {
return async (dispatch) => {
const res = await axios.get(url);
dispatch(setList(res.data.data.channels))
}
}
export { fetchList };
src/App.js
js
import { useDispatch, useSelector } from 'react-redux'
import { fetchList } from './store/modules/listStore'
import { useEffect } from 'react'
function App () {
const { list } = useSelector((state) => state.list)
const dispatch = useDispatch()
useEffect(() => {
dispatch(fetchList())
}, [dispatch]);
return (
<div>
<ol>
{list.map((item) => (<li key={item.id}>{item.name}</li>))}
</ol>
</div>
);
}
export default App;